Events
The forms you create in Designer are based on an event-driven programming model. As you have seen in this chapter, the scripts you write are triggered by events. Events are occurrences or actions that change the state of a form. When these events occur, the scripts behind the events are executed. Because this event-driven model determines how your scripts will perform, you need to consider which events to use, when the events will fire, and how often the events will fire. Let's take a look at two examples.
Examples of How Events Work
The following two examples show you important concepts about events. The first example shows you that multiple events fire based on a single action. The second example shows you that some events can be fired multiple times.
One action fires multiple events
Open the multipleEventsFire.pdf form in Designer. Select Preview PDF to see how a single user action can fire multiple events. Even before you click anything, the form rendering and loading is firing multiple events. Once you click on a form object, you will see how even a small action fires multiple events. Experiment with the example and notice the following:
- The calculate, validate, ready:layout events fire often.
- The initial form rendering and loading fires the initialize, calculate, validate, ready:form, calculate, validate, ready:layout, and docReady events.
- Clicking the Button fires the mouseEnter, mouseDown, enter, mouseUp, click, and mouseExit events (Figure 4.25).
Figure 4.25 Events that are fired when the Button object is clicked.
- Tabbing out of a Text Field fires the exit event of the Text Field and the enter event of the Drop-down List.
- Selecting an item in the Drop-down List fires the mouseDown, enter, preOpen, mouseUp, click, and mouseExit events.
An event can fire multiple times
This example uses a simple calculation script on a Numeric Field's calculate event. You will render the form as a print form and as an interactive form, and see how the calculate event fires twice on an interactive form.
- Open a blank form in Designer and add a Numeric Field to your form. Set the default value of the Numeric Field to zero. You can set this property on the Value tab of the Object palette.
- Select File > Form Properties > Defaults and make sure your preview type is set to Print Form and your default language is set to JavaScript.
- Add the following code to the calculate event of the text field:
NumericField1.rawValue = NumericField1.rawValue + 1;
- Select Preview PDF. Notice that the value of the Numeric Field is one, so you know that your script executed once and the calculate event fired once.
- Go back and change your form's Preview Type to Interactive Form. Select File > Form Properties > Defaults to make this change.
- Select Preview PDF. Notice that the value of the Numeric Field is now two. But you didn't change your script. The different result occurred because the calculate event fired twice during the initial rendering of your interactive form. Each time the event fired, the script ran, so your new result is two.
Types of Events
There are three categories of events in XFA forms: process events, interactive events, and application events. Most of your scripts will run in the interactive events but the process and application events will also be useful to you.
Process events
Process events fire automatically based on an internal XFA process or in response to the firing of an interactive event. The following is a list of the process events in XFA forms:
- calculate
- form:ready
- indexChange (introduced in Designer 8)
- initialize
- layout:ready
- validate
As illustrated in Figure 4.26, process events are fired following a major form change like the form merging process and the form layout process.
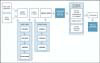
Figure 4.26 The general flow of process events leading up to the PDF form opening in the application. The process events are in bold type. The last event, docReady, is an application event.
Process events are also fired after interactive events are fired. As you saw in the multipleEventsFire.pdf example, a Button click event also fires the layout:ready event for each object on the form. Process events can fire multiple times based on the situation. For instance, in the "An event can fire multiple times" section your calculation script ran twice when you selected Preview PDF. This is because your script ran in the calculate event, which fires twice when an interactive form is opened (Figure 4.26).
Interactive events
Interactive events are very useful for scripting because they fire as a direct result of a user's action. The following is a list of the interactive events in XFA forms:
- change
- click
- enter
- exit
- mouseDown
- mouseEnter
- mouseUp
- preOpen
In the example in the "Getting and setting properties" section earlier in this chapter, you saw how you can retrieve the new text and previous text values when something changes in a Drop-down List:
---- order.#subform[0].shirtSize::change: - (JavaScript, client) --- currentShirtSize.rawValue = xfa.event.newText; previousShirtSize.rawValue = xfa.event.prevText;
This script runs on the change event of the Drop-down List, which is noted in the header section of the script.
Application events
Application events fire as a result of the actions of a client application like Acrobat or a server application like LiveCycle Forms. The following is a list of the application events in XFA forms:
- docClose
- docReady
- postPrint
- postSave
- prePrint
- preSave
- preSubmit
As an example, the postPrint event fires immediately after Acrobat or Reader has sent the form to a printer or spooler. You can add the following script to the postPrint event of your form object to provide instructions to the user about mailing or faxing the printed form:
---- form1::postPrint - (JavaScript, client) -------------------------- xfa.host.messageBox("Instruct the user on where to mail or fax the printed form.");