Debugging Scripts
The debugging tools in Designer keep improving with each version. If you come from a programming background, you may find that these tools are not yet on the level of the debugging tools in IDEs (integrated development environments) like Microsoft's Visual Studio. However, Designer does provide the following features and tools to help you track down problems with your forms and scripts.
The Warnings Tab of the Report Palette
The Warnings tab of the Report palette should be the first place you go to examine the behavior of your form. Designer logs useful information about your form to the Warnings tab when you save your file or when you select Preview PDF. The important information includes missing font information and missing image information (Figure 4.28).
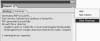
Figure 4.28 The Warnings tab shows that the form has a missing image. You can clear the warning messages by selecting Clear Warnings from the Report menu.
Syntax checking
In Designer ES, the Warnings tab also displays some scripting syntax errors. You can test the syntax of your scripts by clicking the Check Script Syntax icon in the Script Editor. For instance, in the script illustrated in Figure 4.29, Designer highlights the syntax error in the Script Editor and also provides the following description on the Warnings tab:
Error: Too many closing braces, Line:9

Figure 4.29 The syntax checking feature of Designer ES highlights the extra closing brace in the script.
Target versioning
If you are working in Designer ES, you can target a form to a specific version of Acrobat or Reader. If you use a feature in Designer ES that is not available in your targeted client application, you will receive a warning message and a description of the incompatibility on the Warnings tab.
For instance, you can set your form's target version to "Acrobat and Adobe Reader 7.0.5 or later" on the Defaults tab of the Form Properties dialog box (Figure 4.30).

Figure 4.30 The Target Version setting in the Form Properties dialog box of Designer ES.
Now select the Duplex Flip Long Edge option on the PDF Print Options tab of your Form Properties dialog box. Because this is a printing feature that only became available in LiveCycle ES, it will not be compatible with your target version of Acrobat and Reader 7.0.5. Designer will display the following message on the Warnings tab:
The PDF Print Options selected in Form Properties require the form be saved as Acrobat 8.1 or later.
The JavaScript Debugger
Another useful tool for debugging your scripts is the JavaScript Debugger that is included with Adobe Acrobat Professional. To enable the JavaScript debugger for LiveCycle Designer, you must first enable JavaScript and the JavaScript debugger in Acrobat Professional:
- Launch Acrobat Professional and select Edit > Preferences.
- Select JavaScript from the list on the left.
- Select Enable Acrobat JavaScript if it is not already selected.
- On the JavaScript Debugger panel, select "Enable JavaScript debugger after Acrobat is restarted."
- Select "Enable interactive console."
- Select "Show console on errors and messages."
- Click OK to close the Preferences dialog box.
- Quit Acrobat Professional.
- In LiveCycle Designer, click the Preview PDF tab.
- Press Ctrl+J to open the JavaScript Debugger.
Once enabled, the JavaScript Debugger automatically pops up at runtime when an erroneous script is executed (Figure 4.31). In cases where you would like the debugger to be open before running any scripts, you can also press Ctrl+J at runtime to open the debugger.
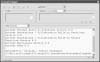
Figure 4.31 The JavaScript Debugger launches when there is a problematic script.
The debugger displays information about what went wrong including the full path of the field containing the erroneous script, the event where the script is located, the line number of the script, and a basic explanation of the error.
The Message Box Method
A practical and useful method of tracking your script's performance at runtime is to use the messageBox method of the host object. The trick is to position your messageBox script at the appropriate point during runtime to retrieve the information you need. For a hypothetical example of using the messageBox method for debugging, open the dataDOM.pdf file from the Samples folder.
In a previous example, you used the buttons on this form to cycle through a series of colors on a Rectangle object. But what if you ran this form and the rectangle did not change colors when you clicked the Next button (Figure 4.32)?
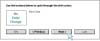
Figure 4.32 If the Next button does not produce a color change, you need to debug your form.
A good place to start debugging this problem is to see if the color value is changing when the Next button is clicked. You can check this value at runtime by adding a messageBox to the appropriate spot in your script:
if (xfa.dataWindow.recordsAfter > 0) { xfa.dataWindow.moveCurrentRecord(1); xfa.host.messageBox("The color is: " + entry.shirtColor.rawValue, "Debugging", 3) } else { app.alert("This is the last record in the data file.");
This script produces the messageBox shown in Figure 4.33, which indicates that your script is retrieving a new color value each time the Next button is clicked. The problem must lie elsewhere.

Figure 4.33 The messageBox proves that your script is receiving a new color value at runtime.
You can also use the JavaScript app alert method to display message boxes at runtime:
app.alert(variable);